Popovers are commonly positioned relative to their invoker (if they have one). When we use the popover attribute, anchoring is tricky, as these popovers are in the top layer, away from the context of their invoker. What options do we have?
Basically, there are two ways to position popovers: one you can use today and one that will be available in the future. I'll detail them below, but first, let's look at why we can't use absolute positioning relative to a container shared by the invoker and the popover.
Not all popovers are anchored, but I expect anchored popovers to be among the most common ones. For popovers that are not anchored, such as toast-like elements, “bottom sheets” or keyboard-triggered command palettes, these positioning constraints do not apply.
Examples of anchored popovers: map toggletip (Extinction Rebellion), date picker (European Sleeper), colour picker (Microsoft Word web app)
See also my other posts on popovers:
- Dialogs and popovers seem similar. How are they different
- Semantics and the popover attribute: what to use when?
- On popover accessibility: what the browser does and doesn't do (with Scott O'Hara)
Top layer elements lose their positioning context
One of the unique characteristics of popovers (again, the ones made with the popover attribute, not just any popover from a design system), is that they get upgraded to the top layer. The top layer is a feature drafted in CSS Positioning, Level 4. The top layer is a layer adjacent to the main document, basically like a bit like a sibling of <html>
.
Some specifics on the top layer:
- It's above all
z-index
es in your document, top layer elements can't usez-index
. Instead, elements are stacked in the order they are added to the top layer. - As developers, we can't put elements in the top layer directly, as it is browser controlled. We can only use certain elements and APIs that then trigger the browser to move an element to the top layer: the Full Screen API,
<dialog>
s withshowModal()
andpopover
'ed elements, currently. - Top layer elements, quoting the specification, “don't lay out normally based on their position in the document”.
When I positioned my first popover, I tried (and failed): I put both the popover and its invoking element in one element with position: relative
. Then I applied position: absolute
to the popover, which I hoped would let me position relative to the container. It didn't, and I think the last item above explains why.
In summary, elements lose their position context when they are upgraded to the top layer. And that's okay, we have other options.
Option 1: position yourself (manually or with a library)
The first option is to position the popover yourself, with script. Because the fact that the top layer element doesn't know about the non-top layer element's position in CSS, doesn't mean you can't store the invoker's position and calculate a position for the popover itself.
There are some specifics to keep in mind, just like with popovers that are built without the popover
attribute: what happens when there's no space or when the popover is near the window? Numerous libraries can help with this, such as Floating UI, an evolution of the infamous Popper library.
Let's look at a minimal example using Floating UI. It assumes you have a popover in your HTML that is connected to a button using popovertarget
:
<button popovertarget="p">Toggle popover</button>
<div id="p" popover>… popover contents go here</div>
By default, browsers show the open popover in the center of the viewport:
The popover is centered
The reason that this happens is that the UA stylesheet applies margin: auto
to popovers. This will reassign any whitespace around the popover equally to all sides as margins. That checks out: if there's the same amount of whitespace left and right, it element will effectively be in the center horizontally (same for top and bottom, but vertically).
For anchored popovers, we want the popover to be near the button that invoked it, not in the center. Let's look at a minimal code example.
In your JavaScript, first import the computePosition
function from @floating-ui
:
import { computePosition } from '@floating-ui/dom';
Then, find the popover:
const popover = document.querySelector('[popover]');
Popovers have a toggle
event, just like the <details>
element, which we'll listen to:
popover.addEventListener('toggle', positionPopover);
In our positionPopover
function, we'll find the invoker, and then, if the newState
property of the event is open
, we'll run the computePosition
function and set the results of its computation as inline styles.
function positionPopover(event) {
const invoker = document.querySelector(`[popovertarget="${popover.getAttribute('id')}"`);
if (event.newState === 'open') {
computePosition(invoker, popover).then(({x, y}) => {
Object.assign(popover.style, {
left: `${x}px`,
top: `${y}px`,
});
});
}
}
To make this work, I also applied these two style declarations to the popover:
margin: 0
, because the UA's auto margin's whitespace gets included in the calculation, with0
we remove that whitespaceposition: absolute
, because popovers getposition: fixed
from the user agent stylesheet and I don't want that on popovers that are anchored to a button
It then looks something like this:
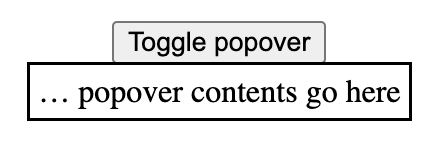
See it in action: Codepen: Positioning a popover with Floating UI.
In the Codepen, I also use some Floating UI config to position the popover from the left. In reality, you probably want to use more of Floating UI's features, to deal with things like resizing (see their tutorial).
Option 2: with Anchor Positioning
To make all of this a whole lot easier (and leave the maths to the browser), a new CSS specification is on the way: Anchor Positioning, Level 1. It exists so that:
a positioned element can size and position itself relative to one or more "anchor elements" elsewhere on the page
This, as they say, is rad, because it will let the browser do your sizing and positioning maths (even automatically- update 4 May 2024: looks like automatic anchoring was removed). It is also exciting, because it doesn't care where your elements are. They can be anywhere in your DOM. And, important for popovers, it also works across the top layer and root element.
Though popovers would get implicit anchoring, you can connect a popover with its invoker via CSS. To find out how all of this works in practice, I recommend Jhey Tompkins's great explainer on Chrome Developers (but note it's currently somewhat outdated, the editor's draft spec changed since that post, and has new editors). Roman Komarov covers his experiments and some interesting use cases in Future CSS: Anchor Positioning, and also wrote Anchor Positioning on 12 days of web.
The Anchor Positioning spec was recently updated, and is currently in the process of being implemented in browsers, hence the Option 1 in this article. But, excitingly, it is in the works. Chromium has already issued an intent to ship anchor positioning, and so did Mozilla/Gecko. The recent updates are still pending TAG review.
Wrapping up
So, in summary: if your popover needs to be anchored to something, like a button or a form field, you can't “just” use absolute positioning. Instead, you can use JavaScript (today), or, excitingly, anchor positioning (in the near-ish future, an Editor's Draft in CSS was published last year and a new version of that with new editors was released in April 2024.
List of updates
- 6 May 2024: Added that Gecko intents to ship anchor positioning.
- 4 May 2024: Reworded to reflect that the editor's draft of the anchor positioning spec was updated (as editor's drafts are), is now different and not yet passed TAG review.
Comments, likes & shares (67)
sim, Derek P. Collins, Ash, Michelle Barker, Roel Groeneveld, Nicolas Chevobbe, Mike Aparicio, James Basoo, Roma Komarov, Wolfr, Mikhail Shornikov, Thomas Broyer, carmya, Martín Baldassarre, Paul Mason, Heather Buchel, dovyden, Curtis Wilcox, Florian Geierstanger, Philip Zastrow, Cory :prami_pride_demi:, Vincent Valentin, Scott Kellum :typetura:, Jason Lawton :wordpress:, Nic, Maïa ????, s:mon, Patrick Grey, Andy Davies, Konnor Rogers, Masataka Yakura, Ollie Boermans and Zacky Ma :favicon: liked this
Jeroen Zwartepoorte, Manuel Matuzović, ~/j4v1, Nicolas Chevobbe, Roma Komarov, Wolfr, Bramus, Thomas Broyer, Bhupesh Singh, Ted M. Young, Ryan Trimble and Konnor Rogers reposted this